All changes will be made in separate extension. It lets you to avoid the conflicts with upcoming extension updates.
1. Create new module for custom changes
Our new module will be called Magetrend_Custom and all files will be located under app/code/Magetrend/Custom/
Create the following files:
app/code/Magetrend/Custom/registration.php
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Magetrend_Custom',
__DIR__
);
app/code/Magetrend/Custom/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../lib/internal/Magento/Framework/Module/etc/module.xsd">
<module name="Magetrend_Custom" schema_version="2.0.0" setup_version="2.0.0">
<sequence>
<module name="Magetrend_PdfTemplates"/>
</sequence>
</module>
</config>
2. Create observer and add new variable
Our extension have already prepared hooks, so all we have to do is to create observer. There are a few events:
- magetrend_pdf_templates_add_additional_data - for all pdf types (order, invoice, shipment, creditmemo)
- magetrend_pdf_templates_add_additional_data_order - to add new variable only for order template
- magetrend_pdf_templates_add_additional_data_invoice - to add new variable only for invoice template
- magetrend_pdf_templates_add_additional_data_shipment - to add new variable only for shipment template
- magetrend_pdf_templates_add_additional_data_creditmemo - to add new variable only for credit memo template
In this example we will use general event magetrend_pdf_templates_add_additional_data. To create an observer create the following files
app/code/Magetrend/Custom/etc/events.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="magetrend_pdf_templates_add_additional_data">
<observer name="additional_vars"
instance="Magetrend\Custom\Observer\Vars"/>
</event>
</config>
app/code/Magetrend/Custom/Observer/Vars.php
<?php
namespace Magetrend\Custom\Observer;
use Magento\Framework\Event\Observer;
class Vars implements \Magento\Framework\Event\ObserverInterface
{
public function execute(Observer $observer)
{
$order = $observer->getSource();
if (!$order instanceof \Magento\Sales\Model\Order) {
$order = $order->getOrder();
}
$customerId = $order->getCustomerId();
if ($customerId > 0) {
$observer->getVariableList()->setData('c_id', 'C-00'.$customerId);
} else {
$observer->getVariableList()->setData('c_id', '');
}
}
}
3. Install new module and test
Connect to your ssh client and run the following commands:
php -f bin/magento setup:upgrade;
php -f bin/magento setup:di:compile;
php -f bin/magento setup:static-content:deploy -f;
After that you should see new variable called c_id (which stands for customer id) in variable list.
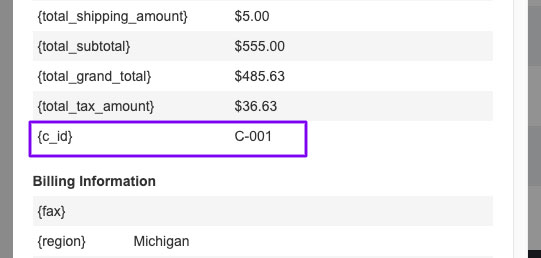