All the changes will be made in a separate extension. That lets you to avoid the conflicts with upcoming updates.
1. Create new module for custom changes
Our new module will be called Magetrend_Custom and all files will be located inside app/code/Magetrend/Custom/ directory
Create the following files:
app/code/Magetrend/Custom/registration.php
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Magetrend_Custom',
__DIR__
);
app/code/Magetrend/Custom/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../../../../lib/internal/Magento/Framework/Module/etc/module.xsd">
<module name="Magetrend_Custom" schema_version="2.0.0" setup_version="2.0.0">
<sequence>
<module name="Magetrend_PdfTemplates"/>
</sequence>
</module>
</config>
2. Add new column configuration
In our extension the columns configuration is stored at config.xml file of extension. To add new column we need to extend this configuration. The first step is to create a config.xml file.
app/code/Magetrend/Custom/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../../Store/etc/config.xsd">
<default>
<pdftemplates>
<items>
<columns>
<author>
<label>Author</label>
<renderer>Magetrend\PdfCustom\Model\Renderer\Author</renderer>
<sort_order>23</sort_order>
<default>
<item_1>S. Jons</item_1>
<item_2>P. Geret</item_2>
<item_3>A. Malone</item_3>
<item_4>D. Geret</item_4>
<item_5>J. Jons</item_5>
</default>
<is_active>
<order>1</order>
<invoice>1</invoice>
<creditmemo>1</creditmemo>
<shipment>1</shipment>
</is_active>
</author>
</columns>
</items>
</pdftemplates>
</default>
</config>
As an example I will add "Author" column where will be printed author of purchased book.
- <author> - unique column name(a-z).
- <renderer> - renderer class which returns value for this column
- <default><item> - default values for items placeholder. Simple text.
- <is_active><order> - here we can specify for which document type column is: order, invoice, shipment, creditmemo
- <sort_order> - integer number according which the columns will be sorted.
3. Create a renderer for column
In the columns configuration we specified that our renderer class is Magetrend\PdfCustom\Model\Renderer\Author. So we have to create the following file
app/code/Magetrend/Custom/Model/Renderer/Author.php
<?php
namespace Magetrend\Custom\Model\Renderer;
use Magento\Framework\Exception\NoSuchEntityException;
class Author extends \Magetrend\PdfTemplates\Model\Pdf\Element\Items\Column\DefaultRenderer
{
public $productRepository;
public function __construct(
\Magetrend\PdfTemplates\Helper\Data $moduleHelper,
\Magetrend\PdfTemplates\Model\Pdf\Element $element,
\Magetrend\PdfTemplates\Model\Pdf\Decorator $decorator,
\Magento\Framework\App\Config\ScopeConfigInterface $scopeConfig,
\Magento\Catalog\Api\ProductRepositoryInterface $productRepository,
array $data = []
) {
$this->productRepository = $productRepository;
parent::__construct($moduleHelper, $element, $decorator, $scopeConfig, $data);
}
public function getRowValue()
{
try {
$product = $this->productRepository->getById($item->getProductId());
} catch (NoSuchEntityException $e) {
return '';
}
return $product->getAuthor();
}
}
In a renderer class, we need to create a method getRowValue() which would return a value for our column. In this example, author is stored in product object, so using product_id we had to load a product object and extract the data from there.
4. Install new module and test
Connect to your ssh client and run the following commands:
php -f bin/magento setup:upgrade;
php -f bin/magento setup:di:compile;
php -f bin/magento setup:static-content:deploy -f;
After that you should see new column added to your pdf template.
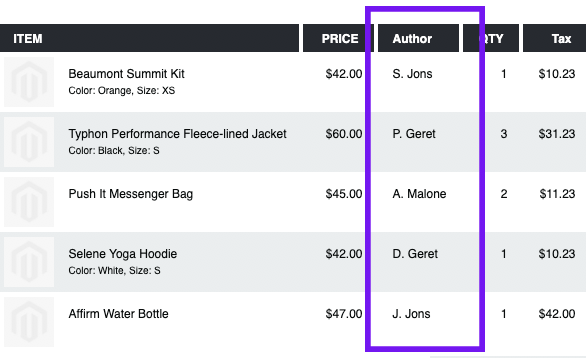